Signal Slot Qt Qml
I am confused now.I did it in Qt 5.2 in the way the code below shows. And the result was that connection( c signal to QML function ) worked well,but connection(QML signal to C slot) didn't work.Did I ignore something important,I only knew that there were some differences between Qt4's way achieving this and Qt5's.Any tip is appreciated,thanks in advance.Sorry for my poor English. The Signal/Slot Editor. The signal and slot used in a connection can be changed after it has been set up. When a connection is configured, it becomes visible in Qt Designer's signal and slot editor where it can be further edited. You can also edit signal/slot connections by double-clicking on the connection path or one of its labels to display. The Cascades framework uses signals and slots to allow objects to communicate with each other. This system is based on the signals and slots mechanism that the underlying Qt development framework uses for inter-object communication. The signals and slots mechanism is how Cascades manages event handling. In other frameworks, you might design. The onMenuClicked slot I defined is simply to output the string that passes through the signal. Note that you have to include QDebug if you want to use the built-in functions of qDebug, qWarning, qCritical, and so on. The slot is prepared, so we need to add a signal to the QML file. The QML file is changed to the following code.
Application and user interface components need to communicate with each other. For example, a button needs to know that the user has clicked on it. The button may change colors to indicate its state or perform some logic. As well, application needs to know whether the user is clicking the button. The application may need to relay this clicking event to other applications.
QML has a signal and handler mechanism, where the signal is the event and the signal is responded to through a signal handler. When a signal is emitted, the corresponding signal handler is invoked. Placing logic such as scripts or other operations in the handler allows the component to respond to the event.
Receiving Signals with Signal Handlers
To receive a notification when a particular signal is emitted for a particular object, the object definition should declare a signal handler named on<Signal> where <Signal> is the name of the signal, with the first letter capitalized. The signal handler should contain the JavaScript code to be executed when the signal handler is invoked.
For example, the MouseArea type from the QtQuick
module has a clicked
signal that is emitted whenever the mouse is clicked within the area. Since the signal name is clicked
, the signal handler for receiving this signal should be named onClicked
. In the example below, whenever the mouse area is clicked, the onClicked
handler is invoked, applying a random color to the Rectangle:
Looking at the MouseArea documentation, you can see the clicked signal is emitted with a parameter named mouse
which is a MouseEvent object that contains further details about the mouse click event. This name can be referred to in our onClicked
handler to access this parameter. For example, the MouseEvent type has x
and y
coordinates that allows us to print out the exact location where the mouse was clicked:
Property Change Signal Handlers
A signal is automatically emitted when the value of a QML property changes. This type of signal is a property change signal and signal handlers for these signals are written in the form on<Property>Changed where <Property> is the name of the property, with the first letter capitalized.
For example, the MouseArea type has a pressed property. To receive a notification whenever this property changes, write a signal handler named onPressedChanged
:
Even though the MouseArea documentation does not document a signal handler named onPressedChanged
, the signal is implicitly provided by the fact that the pressed
property exists.
Using the Connections Type
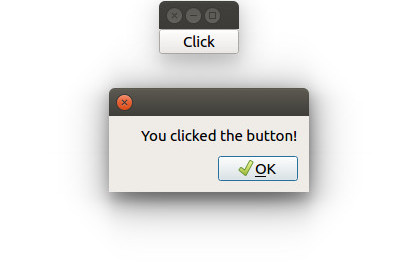
In some cases it may be desirable to access a signal outside of the object that emits it. For these purposes, the QtQuick
module provides the Connections type for connecting to signals of arbitrary objects. A Connections object can receive any signal from its specified target.
For example, the onClicked
handler in the earlier example could have been received by the root Rectangle instead, by placing the onClicked
handler in a Connections object that has its target set to the MouseArea:
Attached Signal Handlers
An attached signal handler is a signal handler that receives a signal from an attaching type rather than the object within which the handler is declared.
For example, Component.onCompleted is an attached signal handler. This handler is often used to execute some JavaScript code when its creation process has been completed, as in the example below:
The onCompleted
handler is not responding to some completed
signal from the Rectangle type. Instead, an object of the Component
attaching type with a completed
signal has automatically been attached to the Rectangle object by the QML engine, and the engine emits this signal when the object is fully created, thus triggering the Component.onCompleted
signal handler.
Attached signal handlers allow objects to be notified of particular signals that are significant to each individual object. If there was no Component.onCompleted
attached signal handler, for example, then an object could not receive this notification without registering for some special signal from some special object. The attached signal handler mechanism enables objects to receive particular signals without these extra processes.
See Attached properties and attached signal handlers for more information on attached signal handlers.
Adding Signals to Custom QML Types
Signals can be added to custom QML types through the signal
keyword.
The syntax for defining a new signal is:
signal <name>[([<type> <parameter name>[, ...]])]
A signal is emitted by invoking the signal as a method.
For example, say the code below is defined in a file named SquareButton.qml
. The root Rectangle object has an activated
signal. When the child MouseArea is clicked, it emits the parent's activated
signal with the coordinates of the mouse click:
Now any objects of the SquareButton
can connect to the activated
signal using an onActivated
signal handler:
See Signal Attributes for more details on writing signals for custom QML types.
Connecting Signals to Methods and Signals
Signal objects have a connect()
method to a connect a signal either to a method or another signal. When a signal is connected to a method, the method is automatically invoked whenever the signal is emitted. This mechanism enables a signal to be received by a method instead of a signal handler.
Below, the messageReceived
signal is connected to three methods using the connect()
method:
In many cases it is sufficient to receive signals through signal handlers rather than using the connect() function. However, using the connect
method allows a signal to be received by multiple methods as shown above, which would not be possible with signal handlers as they must be uniquely named. Also, the connect
method is useful when connecting signals to dynamically created objects.
There is a corresponding disconnect()
method for removing connected signals:
Signal to Signal Connect
By connecting signals to other signals, the connect()
method can form different signal chains.
Whenever the MouseAreaclicked
signal is emitted, the send
signal will automatically be emitted as well.
© 2020 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.
The Cascades framework uses signals and slots to allow objects to communicate with each other. This system is based on the signals and slots mechanism that the underlying Qt development framework uses for inter-object communication.
The signals and slots mechanism is how Cascades manages event handling. In other frameworks, you might design your app to listen for a particular event that you're interested in, such as a touch event. When that event occurs, your app processes the event and responds to it. Cascades uses a similar approach but provides an extra layer (signals and slots) on top of the fundamental event system. This layer makes handling different types of events easier and more intuitive. The idea of signals and slots is different from normal event handling approaches, so throughout this documentation you'll notice that 'signals and slots', instead of 'event handling', is used to describe the system.
In many apps that you create, when a change occurs to one object, you want to notify other objects of the change. The notification takes the form of a signal. A signal is emitted by an object when some type of event occurs, such as when a property of the object changes. Other objects can receive these signals by using slots. A slot is a function that is called when an object receives a signal. Slots are member functions of a class, and can either be called directly or be connected to a signal. In Cascades, there are many predefined signals that you can connect to slots in your app, but you're also free to create your own signals and slots if you need them.
For example, the Button control includes a predefined signal called clicked(), which (as you'd expect) is emitted when the button is clicked. You can connect this signal to another object in your app to respond to the signal when it's emitted. The Slider control includes a slot called resetValue(), which resets the value of the slider to its default value. You could connect the button's clicked() signal to the slider's resetValue() slot so that when the button is clicked, the slider's value is reset.
The emission of signals from objects is separate and distinct from the connection of signals to slots; signals are emitted from objects even if no slots are connected to those signals. Also, a signal-to-slot connection isn't necessarily a one-to-one relationship. You can connect many signals to one slot, or one signal to many slots. You can also connect signals to other signals, so that when one signal is emitted, the connected signal is also emitted.
For more detailed information, see Signals & Slots on the Qt website. The use of signals and slots in Cascades is similar to the implementation in Qt. There are some differences, and the following sections describe how to use the specific implementation in Cascades.
Using predefined signals
Most Cascades UI controls include predefined signals that are emitted when something interesting happens to the control. A button emits a signal when it's clicked, a slider emits a signal when its value changes, and a text area emits a signal when its text changes. You can respond to these signals in either QML or C++.
Responding to predefined signals in QML
In QML, predefined signals have corresponding signal handlers that are created automatically for your use. The names of these signal handlers are based on the signal that they correspond to, and start with the prefix 'on'. For example, the clicked() signal for a Button has an onClicked signal handler. Notice that the casing of the signal name changes to conform to the lower camel case format (from clicked to onClicked).
Inside the signal handler, you can use JavaScript to define how the control should respond to the signal. You can change the control's property values, change other controls' property values, call functions, and so on. Here's how to create a button that changes its text when it's clicked:
Some predefined signals include parameters that you can access and use in the signal handlers. These parameters provide additional information about the change that occurred. You can check the API reference to determine whether a signal provides parameters and what they are.
For example, the CheckBox control emits a checkedChanged() signal when its checked state changes (checked or cleared). This signal includes a Boolean parameter, checked , that contains the new checked state of the check box. Here's how to create a check box that, when its checked state changes, updates the text of an adjacent Label:
Using predefined signals and their associated signal handlers is an easy way to provide simple interactions in your apps. You can respond to signals from core UI controls with minimal effort and code. The signal handlers are provided for you already, and all you need to do is fill in the actions you want to take.
Responding to predefined signals in C++
In C++, you use the QObject::connect() static function to connect a signal to a slot. This function is overloaded to accept a few different argument combinations, but you typically call the function with the following four arguments:
- An object representing the sender of the signal
- The signal to send
- An object representing the receiver of the signal
- The slot to receive the signal
As an example, consider a smoke detector and sprinkler system in your home or office. When the smoke detector detects smoke, it sends a signal to the sprinkler to indicate that the sprinkler should start dispensing water to put out a potential fire.
You could model this behavior in Cascades by using signals and slots. You might have an object that represents the smoke detector, emitting a signal when it detects smoke. You might also have an object that represents the sprinkler, with a slot that dispenses the water.
Here's how you could connect the smoke detector's signal to the sprinkler's slot:
When you specify the signal and slot in connect(), you need to use the SIGNAL() and SLOT() macros, respectively. These macros convert the names of the signal and slot that you want to connect to string literals that are used by connect().
It's easy to mistype signal or slot names when using connect(), or put parentheses in the wrong locations, so it can be a good idea to check the return value from connect() to make sure that there aren't any errors. The connect() function returns a Boolean value that indicates whether the connection of the signal and slot was successful. You can use Q_ASSERT() to test the return value and generate a warning message if the connection isn't successful.
Here's how to check the return value from connect() and generate a warning if something's gone wrong with the connection:
In C++, you need to be explicit when handling signals. You can't use the signal handlers for predefined signals the way you can in QML. Instead, to handle a predefined signal that you're interested in, you have two choices: you can connect the signal to an existing slot of another class, or define your own slot to handle the signal and then connect the signal to that slot.
When you connect a signal to an existing slot of another class, you usually pass some information directly from the signal object to the slot object. This information is passed using signal parameters. For example, the Slider control has a signal called immediateValueChanged() that's emitted when the value of the slider changes. This signal includes a value parameter that contains the new value of the slider. You could connect this signal to the setPreferredWidth() slot of a Container, and use the parameter to have the width of the container change as the value of the slider changes. Here's how to do that:
To use this approach to pass information between controls, the signal and the slot need to use the same types of parameters. You can't connect a signal with a float parameter to a slot that expects a QString parameter. Even though the types of parameters must match, the number of parameters doesn't need to. The slot can have the same number of parameters (or fewer) as the signal that it's connected to. For example, you can connect a signal with two parameters (a float and a bool) to a slot with only a float parameter; the additional bool parameter from the signal is ignored.
Connecting signals to existing slots works well for simple connections, but what if the signal and slot parameters don't match, or you need to perform a more complex task in response to the signal? In these cases, you can define your own slot to handle the signal.
When you create a slot, you're simply creating a member function for the class you're working with. To allow the function to act as a slot, you place the function declaration in a public slots: section in the class header file:
You can then define the function in the corresponding source file, and connect a signal to the slot using QObject::connect(). Slot functions almost always have a return type of void. You could create a slot that returns a value or an object, but the signals and slots mechanism wouldn't allow you to access this return value.
In one of the code samples above, QML was used to change the text of a Label when the associated check box changed its state. Here's how to achieve the same result using a custom slot in C++:
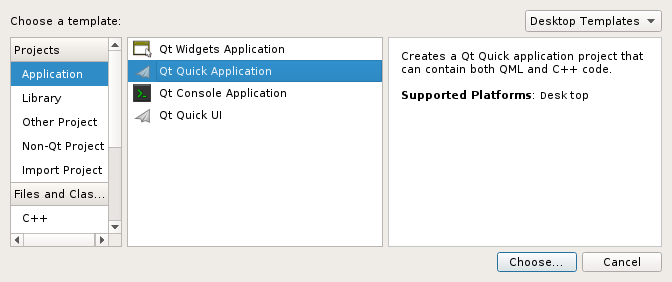
Although using C++ to create your own slots involves more code, it's also more flexible. You can do more complex tasks in your slot implementations, and you can use the full range of C++ functionality instead of being limited only to JavaScript.
Creating your own signals

If the predefined signals in Cascades aren't sufficient for your app, or if you want to emit signals from custom objects that you create, you can create your own signals and connect them to slots in either QML or C++.
Creating signals in QML
In QML, you create a custom signal for a control by using the keyword signal and providing any parameters that the signal includes when it's emitted:
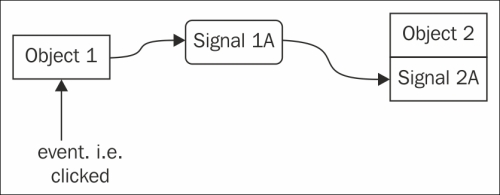
Similar to predefined signals, Cascades creates signal handlers for custom signals automatically. The custom signal mySignal() above has a signal handler called onMySignal, and the parameters value and enabled are available for you to use in the body of the signal handler.
To emit a custom signal, you simply call the signal the same way you call a regular function. Here's how to create a button that, when it's clicked, emits a custom signal called clickedWithText. This signal includes a string parameter called currentText, which contains the text of the button:
Qt Qml Signal Slot Example
In addition to using the signal handler that Cascades creates for you, you can create your own slot and connect a custom signal to it. You define a slot as a JavaScript function, and connect your signal to that slot. Here's how to create a button that, when it's clicked, displays its text in a text area:
There are other approaches you could use to achieve this result. You could decide to create your button and text area as separate QML components, and define each in their own .qml file. For more information about creating custom components, see Custom QML components.
Creating signals in C++
In C++, you can create a custom signal by placing the declaration in a signals: section in the class header file. You can also include any parameters that the signal includes when it's emitted:
Like slots, signals almost always have a return type of void. When you want to emit a signal, you use the keyword emit:
Qt Signal Slot C++ Qml
You can connect a custom signal to a slot by using QObject::connect(), in the same way that you connect a predefined signal to a slot:
Here's one way to create the same custom signal from one of the QML code samples above, in C++:
As with the equivalent QML code sample, there are several other ways that you could achieve this result, such as creating custom components for each control.